Muting DSTV using Arduino
by skullkey

I have been playing around with infrared remote controls as part of the upcoming Introduction to MSP430 course – Guy and I were looking for an interesting use of PWM and timers, so the suggestion was to produce an infrared signal using an IR led.
I set out to learn a bit more about IR and prototype using the Arduino. After searching online, I found Ken Shirriff’s excellent IR library for Arduino on Github: https://github.com/shirriff/Arduino-IRremote
It supports some standard TV remote controls and a “raw” mode which looked promising for controlling other things, e.g. my DSTV remote control, air conditioner etc
In this mode you can give it an array of unsigned integers. For example:
unsigned int raw[36]={250,842,242,2374,...,250,81862};
For background on how IR remote controls works check out this article on the irq5 blog. In short the signal is encoded in bursts of “on” and “off”. When the LED is on, it is PWM’d at a certain frequency. To use the “raw” mode, we need to know the PWM frequency (typical values between 35-40 kHz) and then the “on” and “off” durations. The array is then made as follows:
unsigned int raw[36]={"duration on","duration off",....};
Using an IR LED, a resistor (e.g. 10K) and an oscilloscope, one can readily discover the signal sent from the remote control:
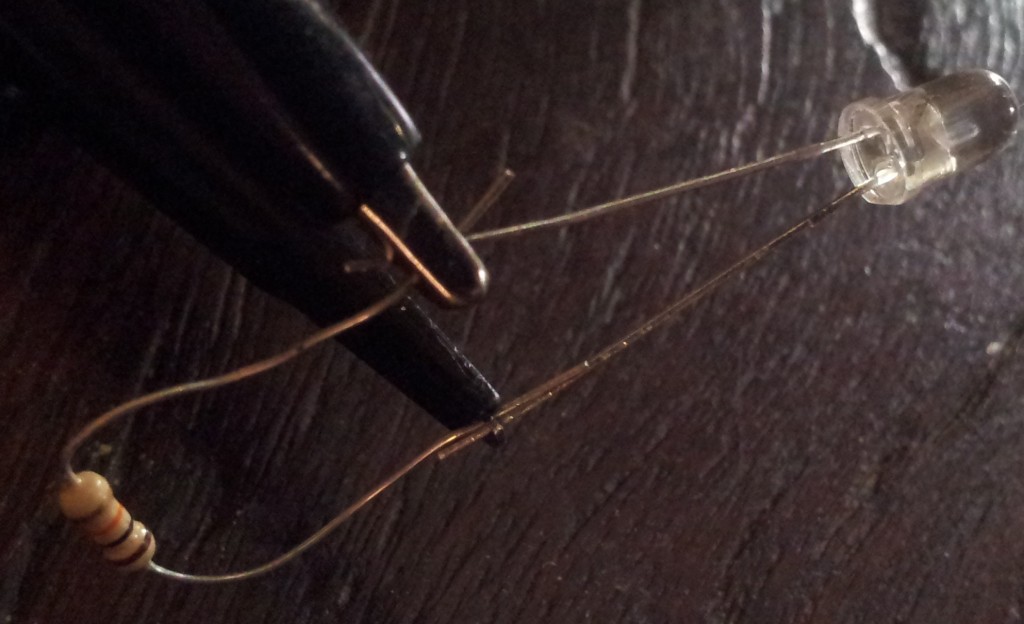
One "ON" pulse showing PWM The duration of seven modulation cycle for the DSTV remote control is measure as 183uS, which corresponds to ~38kHz.
To get the on-off durations, the easiest is to use an IR receiver and the InfraredReceivers sketch found on http://playground.arduino.cc/Code/InfraredReceivers
Infrared receiver Infrared receivers removes the 38kHz and gives a digital HIGH / LOW for the received pulses. The Arduino Playground sketch samples the duration of these pulses at 4uS and prints to the serial console.
However, to use the output I had to subtract 10uS from each measurement – I suspectthis is an additional delay in IRremote library to switch the LED.
Muting DSTV
Connecting an IR LED to pin 3 through a 220 ohm resistor
IR LED connected to pin3 and 220 ohm resistor to ground and running the following sketch mutes DSTV PVR:
#include <IRremote.h> IRsend irsend; void setup(){} unsigned int raw[36]= {250,842,242,2374,250,834,250,2790,250,1254, 250,1258,246,1258,242,1670,250,12938,254,838,250,974,250,698, 242,838,254,694,246,2370,254,698,246,694,250,81862}; void loop() { irsend.sendRaw(raw,36,38); delay(2000); }
Next steps is to apply this to the Airconditioner at House4Hack, to be able to control that as part of the course.
I have been playing around with infrared remote controls as part of the upcoming Introduction to MSP430 course – Guy and I were looking for an interesting use of PWM and timers, so the suggestion was to produce an infrared signal using an IR led. I set out to learn a bit more about IR…
Get involved
Visit us on a Tuesday:
- Join us at 4 Burger Ave, Lyttleton Manor, Centurion every Tuesday evening from 18.00 till late (bring a project to work on or beer)
Telegram Group:
- Send a message to Schalk on Telegram to add you to the group - currently >100 users, relevant conversations for makers
IRL:
- Schalk 082 777 7098
After hours only, please rather send telegram message - Toby
http://tobykurien.com
- Not active at the moment, if you are interested in presenting a course, please let Schalk know